Have you ever needed to convert a number written out in words (like “one hundred twenty-three”) to an integer in a Java program? As a passionate Java programmer, I was thrilled when I discovered how to do this using some simple string manipulation and built-in methods!
In this post, I’ll share the step-by-step approach to alphabet to integer conversion in Java. I’m excited to walk through this incredibly useful technique with you!
Let’s dive in and see how it works!
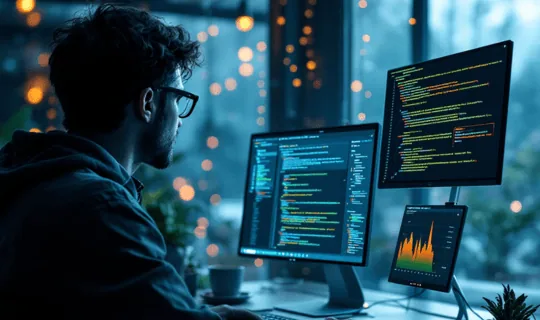
Understanding Java’s String and Integer Handling
To convert alphabetic numbers to integers, we first need to understand how Java handles strings and integers.
Java strings are objects that represent sequences of characters. We can manipulate them using handy methods like split()
to break the string into words. This helps parse our numeric text input.
Java integers, on the other hand, are primitive data types that store whole number values. We ultimately want to convert our string to an int. Helpful methods like Integer.parseInt()
make this easy.
Now let’s look at the step-by-step process…
Mapping Number Words to Integers
The first step is to create a mapping of all the number words (“one”, “two”, “hundred”, etc) to their integer values.
We can use a HashMap to store this mapping in Java:
Map<String, Integer> numberMapping = new HashMap<>();
numberMapping.put("zero", 0);
numberMapping.put("one", 1);
numberMapping.put("two", 2);
//...
numberMapping.put("hundred", 100);
numberMapping.put("thousand", 1000);
This gives us an easy way to lookup the integer for a given number word!
Parsing and Processing the Input
Next, we need to split the input string into individual words and process each word to generate the final integer:
String input = "one hundred twenty three";
String[] words = input.split(" ");
The split()
method breaks the string on spaces, giving us an array of words.
We can then iterate through the words, use our mapping to find the corresponding integer, and sum upthe values:
int result = 0;
for (String word : words) {
result += numberMapping.get(word);
}
// result = 123
Handling Special Cases
There are some special cases we need to handle, like “hundred” and “thousand”:
- For “hundred”, we multiply the current value by 100
- For “thousand”, we multiply the current value by 1000 and add it to the result
if (word.equals("hundred")) {
currentValue *= 100;
}
else if (word.equals("thousand")) {
result += currentValue * 1000;
currentValue = 0;
}
This ensures we handle larger numbers correctly!
Putting it All Together
By following the steps we’ve covered:
- Creating a word-to-number mapping
- Parsing the input string
- Iterating through words and summing values
- Handling special cases
We can easily convert alphabetic numbers like “one hundred twenty-three” into their integer equivalent in Java!
Here is the complete code for reference:
Map<String, Integer> map = new HashMap<>();
//populate mapping
String input = "one hundred twenty-three";
String[] words = input.split(" ");
int result = 0;
int currentValue = 0;
for (String word : words) {
//handle special cases
if (word.equals("hundred")) {
currentValue *= 100;
}
else if (word.equals("thousand")) {
result += currentValue * 1000;
currentValue = 0;
}
else {
currentValue += map.get(word);
}
}
result += currentValue;
System.out.println(result); //123
This technique is immensely helpful for processing numeric input given in words, which comes up often in natural language processing. I’m thrilled to have discovered this approach!
Let me know if you have any other Java programming questions. I absolutely love talking about this stuff!